Writing A Twitter Bot in Golang (Part 1)
One small bot for you but one large bot for mankind.
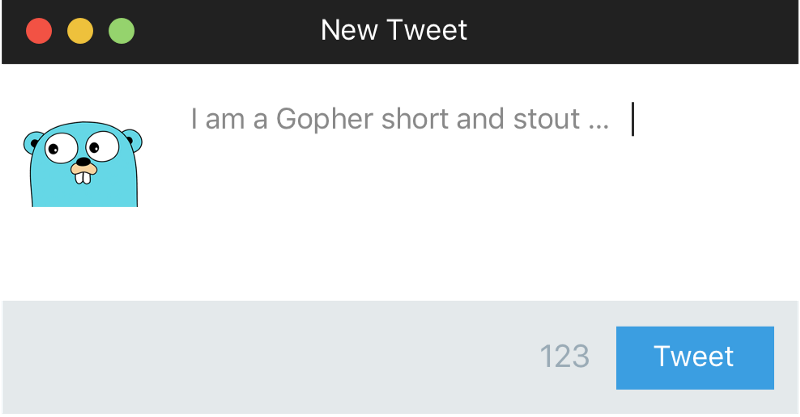
Golang has gained enough popularity in the past few years that it can no longer be avoided. To dive deep into the languageI have started to build a simple twitter bot.
To start with, I decided to keep the problem statement fairly simple:
Search 5 recent tweets with tag #golang and Retweet them.
It took me a couple of hours to hunt for the libraries and some example code to come up with code for this tutorial. You will take no more than 10 minutes if you follow the steps properly.
Before we get started we will need 3 things :
- Twitter API Keys: Go to the link and follow the instructions. (You can also refer to this article if you need help)
- Golang: If you don’t have golang installed already then download it from their website.
- go-twitter: go-twitter is a Go client library for the Twitter API. Check the usage section or try the examples to see how to access the Twitter API.
Setup Workspace
Start with creating a directory and the files that are needed. Also install the dependencies for the project
mkdir -p ~/tweetbot
cd ~/tweetbot
touch bot.go
go get -u
Test API keys
Before getting into implementation of the logic for search and retweet let’s quickly test our twitter-api keys. Make a file name login_test.go
- import required packages.
- Add twitter API secrets. These keys and tokens are sensitive and you should not share it with anybody.
- Initialize twitter
client
verifyParams
variable is required forclient.Account.VerifyCredentials
function.- The
client.Accounts.VerifyCredentials
returnsuser
information. - Print the user’s twitter handle with
user.ScreenName
.
$ go run login_test.go
User's Name:abdulkarim_me
Build The Bot
Our problem statements has 2 parts
- Search 5 recent tweets with tag #golang
- Retweet them.
Search
For search we will use Tweets()
function which returns a collection of Tweets matching a search query. First build the searchParams
with required values and pass it to the Search function. (Only Query
is a required field here, rest can be omitted).
...
// Search tweets to retweet
searchParams := &twitter.SearchTweetParams{
Query: "#golang",
Count: 5,
ResultType: "recent",
Lang: "en",
}
searchResult, _, _ := client.Search.Tweets(searchParams)
...
Retweet
Iterate over the searchResult
and retweet all the tweets for which we will need the tweetID of the tweets. Retweet
retweets the Tweet with the given id and returns the original Tweet with embedded retweet details. Requires a user auth context.
...
// Iterate an retweet
for _, tweet := range searchResult.Statuses {
tweet_id := tweet.ID
client.Statuses.Retweet(tweet_id, &twitter.StatusRetweetParams{})
fmt.Printf("RETWEETED: %+v\n", tweet.Text)
}
...
Voila! That’s it and you have your twitter bot ready that will retweet #golang tweets (or anything you want) for you. The complete code looks like this
$ go run bot.go
RETWEETED: RT @dgryski: The 10 minutes where you test your #golang replacement of a #perl service:
(Y axis is in ms) https://t.co/SB9D2am3aI
RETWEETED: RT @kdnuggets: Understanding #Tensorflow using #GoLang https://t.co/J1dsjZzPKk https://t.co/4Et9pZIiio
RETWEETED: RT @cjrolo: Never imagined that it would be possible that @code is replacing all my IDEs so fast. #golang #python #java even #markdown and…
RETWEETED: RT @cjrolo: Never imagined that it would be possible that @code is replacing all my IDEs so fast. #golang #python #java even #markdown and…
RETWEETED: Never imagined that it would be possible that @code is replacing all my IDEs so fast. #golang #python #java even #markdown and LaTeX...
Our bot is very primitive and will need a lot more attention in the coming days. Here are some of the ToDo’s that I have in mind
- Parameterize the bot. (#golang or #ruby should be passed as an arguement)
- Greet your followers
- Favorite if somebody retweets your tweet
- Follow Bot. (If follow <-> You follow)
- Dockerize the bot so it can be executed on any machine.
- Setup a cronjob